kcl-samples → dual-basin-utility-sink →
dual-basin-utility-sink
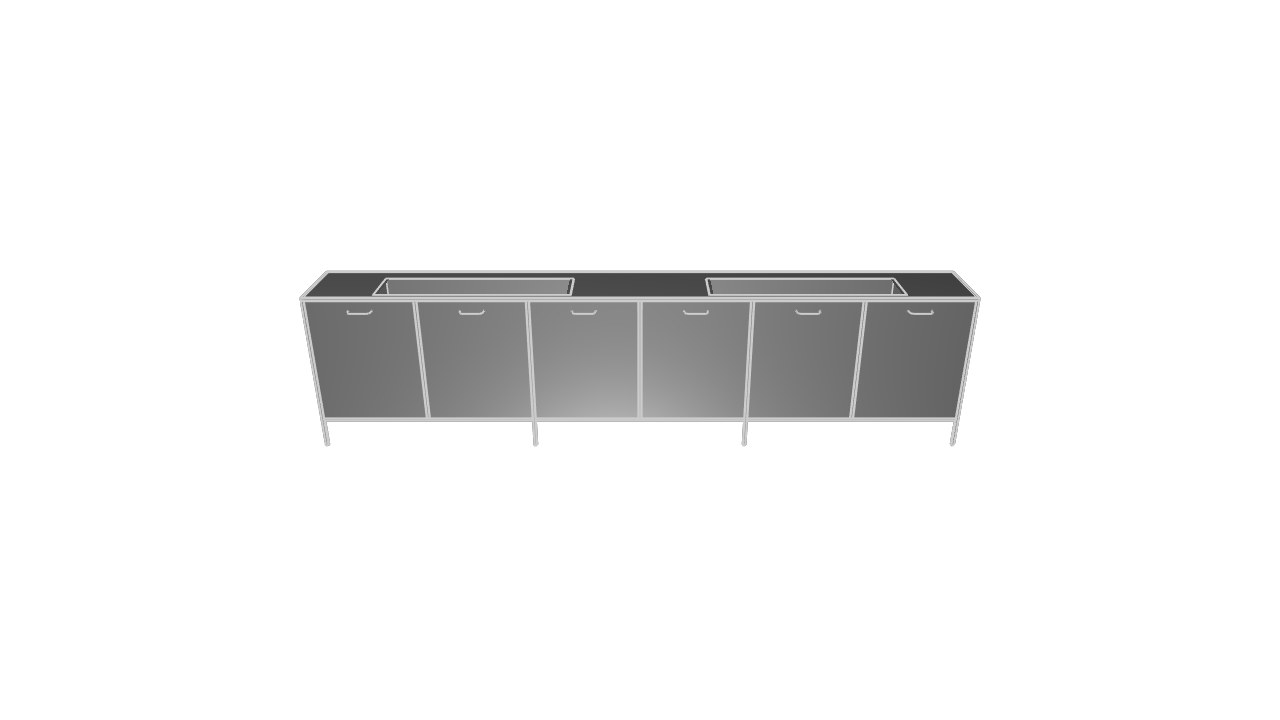
KCL
// Dual-Basin Utility Sink
// A stainless steel sink unit with dual rectangular basins and six under-counter storage compartments.
// Set units
@settings(defaultLengthUnit = mm)
// Define parameters
tableHeight = 850
tableWidth = 3400
tableDepth = 400
profileThickness = 13
metalThickness = 2
blockCount = 3
blockWidth = (tableWidth - profileThickness) / 3
blockHeight = tableHeight - metalThickness - 0.5
blockDepth = tableDepth - profileThickness
blockSubdivisionCount = 2
blockSubdivisionWidth = blockWidth / blockSubdivisionCount
// Geometry
floorPlane = startSketchOn(XY)
// legs
legHeight = blockHeight - profileThickness
legCount = blockCount + 1
legBody = startProfileAt([0, 0], floorPlane)
|> yLine(length = profileThickness)
|> xLine(length = profileThickness)
|> yLine(length = -profileThickness)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> patternLinear2d(axis = [1, 0], instances = legCount, distance = blockWidth)
|> patternLinear2d(axis = [0, 1], instances = 2, distance = blockDepth)
|> extrude(length = legHeight)
// lower belt
lowerBeltHeightAboveTheFloor = 150
lowerBeltLengthX = blockWidth - profileThickness
lowerBeltPlane = startSketchOn(offsetPlane(XY, offset = lowerBeltHeightAboveTheFloor))
lowerBeltBodyX = startProfileAt([profileThickness, 0], lowerBeltPlane)
|> yLine(length = profileThickness)
|> xLine(length = lowerBeltLengthX)
|> yLine(length = -profileThickness)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> patternLinear2d(axis = [1, 0], instances = blockCount, distance = blockWidth)
|> patternLinear2d(axis = [0, 1], instances = 2, distance = blockDepth)
|> extrude(length = profileThickness)
lowerBeltLengthY = blockDepth - profileThickness
lowerBeltBodyY = startProfileAt([0, profileThickness], lowerBeltPlane)
|> yLine(length = lowerBeltLengthY)
|> xLine(length = profileThickness)
|> yLine(length = -lowerBeltLengthY)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> patternLinear2d(axis = [1, 0], instances = 2, distance = tableWidth - profileThickness)
|> extrude(length = profileThickness)
// pillars
pillarHeightAboveTheFloor = lowerBeltHeightAboveTheFloor + profileThickness
pillarPlane = startSketchOn(offsetPlane(XY, offset = pillarHeightAboveTheFloor))
pillarTotalHeight = blockHeight - profileThickness - pillarHeightAboveTheFloor
pillarBody = startProfileAt([blockSubdivisionWidth, 0], pillarPlane)
|> yLine(length = profileThickness)
|> xLine(length = profileThickness)
|> yLine(length = -profileThickness)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> patternLinear2d(axis = [1, 0], instances = blockCount, distance = blockWidth)
|> patternLinear2d(axis = [0, 1], instances = 2, distance = blockDepth)
|> extrude(length = pillarTotalHeight)
// upper belt
upperBeltPlane = startSketchOn(offsetPlane(XY, offset = blockHeight))
upperBeltBodyX = startProfileAt([0, 0], upperBeltPlane)
|> yLine(length = profileThickness)
|> xLine(length = tableWidth)
|> yLine(length = -profileThickness)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> patternLinear2d(axis = [0, 1], instances = 2, distance = blockDepth)
|> extrude(length = -profileThickness)
upperBeltLengthY = blockDepth - profileThickness
upperBeltBodyY = startProfileAt([0, profileThickness], upperBeltPlane)
|> yLine(length = upperBeltLengthY)
|> xLine(length = profileThickness)
|> yLine(length = -upperBeltLengthY)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> patternLinear2d(axis = [1, 0], instances = 2, distance = tableWidth - profileThickness)
|> extrude(length = -profileThickness)
// sink
tableTopPlane = startSketchOn(offsetPlane(XY, offset = tableHeight))
tableTopBody = startProfileAt([0, 0], tableTopPlane)
|> yLine(length = tableDepth)
|> xLine(length = tableWidth)
|> yLine(length = -tableDepth)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> extrude(length = -metalThickness)
sinkCount = 2
sinkWidth = 1000
sinkLength = 250
sinkDepth = 200
sinkOffsetFront = 40
sinkOffsetLeft = 350
sinkSpacing = tableWidth - sinkWidth - (sinkOffsetLeft * 2)
sinkPlaneOutside = startSketchOn(tableTopBody, face = START)
sinkBodyOutside = startProfileAt([-sinkOffsetLeft, sinkOffsetFront], sinkPlaneOutside)
|> yLine(length = sinkLength)
|> xLine(length = -sinkWidth)
|> yLine(length = -sinkLength)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> patternLinear2d(axis = [-1, 0], instances = sinkCount, distance = sinkSpacing)
|> extrude(length = sinkDepth)
sinkPlaneInside = startSketchOn(tableTopBody, face = END)
sinkBodyInside = startProfileAt([
sinkOffsetLeft + metalThickness,
sinkOffsetFront + metalThickness
], sinkPlaneInside)
|> yLine(length = sinkLength - (metalThickness * 2))
|> xLine(length = sinkWidth - (metalThickness * 2))
|> yLine(length = -sinkLength + metalThickness * 2)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> patternLinear2d(axis = [1, 0], instances = sinkCount, distance = sinkSpacing)
|> extrude(length = -sinkDepth)
// door panels
doorGap = 2
doorWidth = blockSubdivisionWidth - profileThickness - (doorGap * 2)
doorStart = profileThickness + doorGap
doorHeightAboveTheFloor = pillarHeightAboveTheFloor + doorGap
doorHeight = blockHeight - doorHeightAboveTheFloor - profileThickness - doorGap
doorCount = blockCount * blockSubdivisionCount
doorPlane = startSketchOn(offsetPlane(XY, offset = doorHeightAboveTheFloor))
doorBody = startProfileAt([doorStart, 0], doorPlane)
|> yLine(length = profileThickness)
|> xLine(length = doorWidth)
|> yLine(length = -profileThickness)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> patternLinear2d(axis = [1, 0], instances = doorCount, distance = blockSubdivisionWidth)
|> extrude(length = doorHeight)
// side panels
panelWidth = blockDepth - profileThickness - (doorGap * 2)
panelCount = doorCount + 1
panelSpacing = tableWidth - profileThickness
panelBody = startProfileAt([0, doorStart], doorPlane)
|> yLine(length = panelWidth)
|> xLine(length = profileThickness)
|> yLine(length = -panelWidth)
|> line(endAbsolute = [profileStartX(%), profileStartY(%)])
|> close()
|> patternLinear2d(axis = [1, 0], instances = 2, distance = panelSpacing)
|> extrude(length = doorHeight)
// handle
handleDepth = 40
handleWidth = 120
handleFillet = 20
handleHeightAboveTheFloor = 780
handleOffset = doorStart + doorWidth / 2 - (handleWidth / 2)
handleLengthSegmentA = handleDepth - handleFillet
handleLengthSegmentB = handleWidth - (handleFillet * 2)
handlePlane = startSketchOn(offsetPlane(XY, offset = handleHeightAboveTheFloor))
handleProfilePath = startProfileAt([0 + handleOffset, 0], handlePlane)
|> yLine(length = -handleLengthSegmentA)
|> tangentialArc(endAbsolute = [
handleFillet + handleOffset,
-handleDepth
])
|> xLine(length = handleLengthSegmentB)
|> tangentialArc(endAbsolute = [
handleOffset + handleWidth,
-handleLengthSegmentA
])
|> yLine(length = handleLengthSegmentA)
handleSectionPlane = startSketchOn(XZ)
handleProfileSection = circle(
handleSectionPlane,
center = [
handleOffset,
handleHeightAboveTheFloor
],
radius = 2,
)
handleBody = sweep(handleProfileSection, path = handleProfilePath)
|> patternLinear3d(axis = [1, 0, 0], instances = doorCount, distance = blockSubdivisionWidth)