kcl-samples → makeup-mirror
makeup-mirror
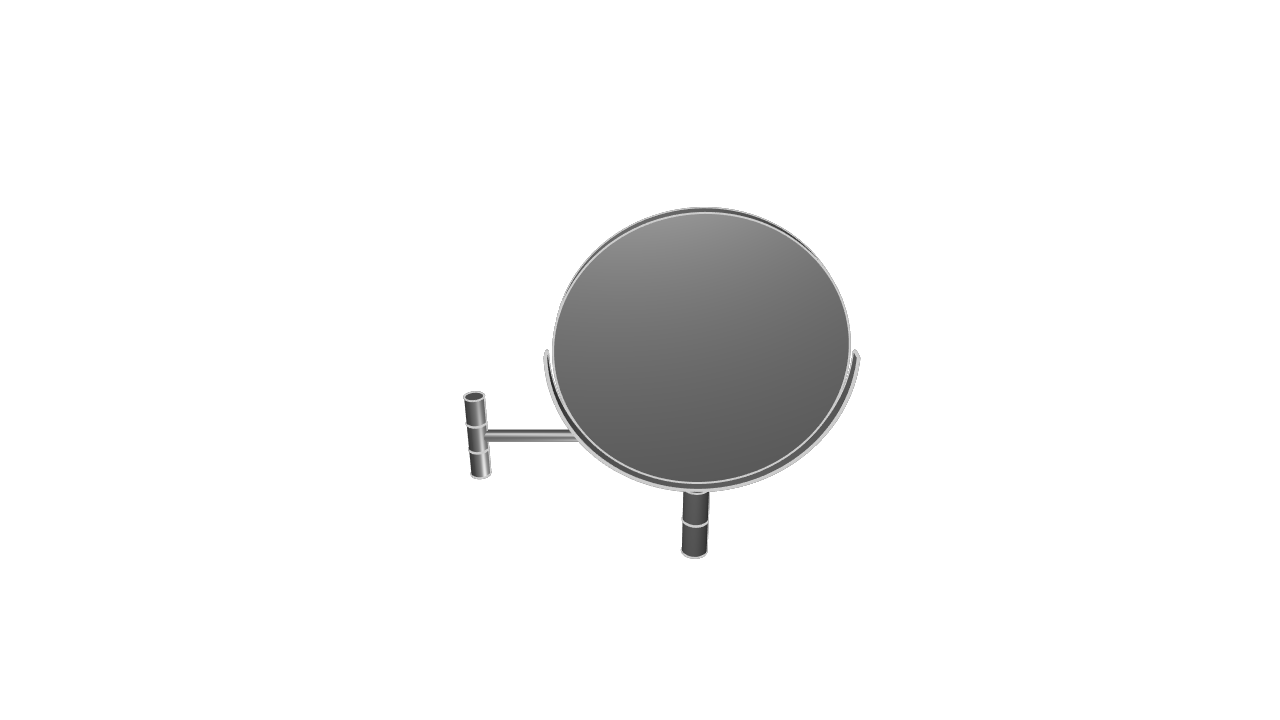
KCL
// Makeup Mirror
// A circular vanity mirror mounted on a swiveling arm with pivot joints, used for personal grooming.
// Set units
@settings(defaultLengthUnit = mm, kclVersion = 1.0)
// Hinge parameters
hingeRadius = 8
hingeHeight = hingeRadius * 3
hingeGap = 0.5
// Arm parameters
armLength = 170
armRadius = 5
// Mirror parameters
mirrorRadius = 170 / 2
mirrorThickness = 10
archToMirrorGap = 5
archThickness = 1
archRadius = mirrorRadius + archToMirrorGap
// Geometry
// Add a function to create the hinge
fn hingeFn(x, y, z) {
hingeBody = startSketchOn(offsetPlane(XY, offset = z))
|> circle(center = [x, y], radius = hingeRadius)
|> extrude(length = hingeHeight)
return hingeBody
}
hingePartA1 = hingeFn(x = 0, y = 0, z = 0)
hingePartA2 = hingeFn(x = 0, y = 0, z = hingeHeight + hingeGap)
hingePartA3 = hingeFn(x = 0, y = 0, z = hingeHeight * 2 + hingeGap * 2)
hingePartB2 = hingeFn(x = armLength, y = 0, z = hingeHeight + hingeGap)
hingePartB3 = hingeFn(x = armLength, y = 0, z = hingeHeight * 2 + hingeGap * 2)
hingePartC2 = hingeFn(x = armLength, y = -armLength, z = hingeHeight * 2 + hingeGap * 2)
hingePartC3 = hingeFn(x = armLength, y = -armLength, z = hingeHeight * 3 + hingeGap * 3)
// Add a function to create the arm
fn armFn(plane, offset, altitude) {
armBody = startSketchOn(plane)
|> circle(center = [offset, altitude], radius = armRadius)
|> extrude(length = armLength)
return armBody
}
armPartA = armFn(plane = YZ, offset = 0, altitude = hingeHeight * 1.5 + hingeGap)
armPartB = armFn(plane = XZ, offset = armLength, altitude = hingeHeight * 2.5 + hingeGap * 2)
// Add a function to create the mirror
fn mirrorFn(plane, offsetX, offsetY, altitude, radius, tiefe, gestellR, gestellD) {
armPlane = startSketchOn(offsetPlane(plane, offset = offsetY - (tiefe / 2)))
armBody = circle(armPlane, center = [offsetX, altitude], radius = radius)
|> extrude(length = tiefe)
archBody = startProfile(armPlane, at = [offsetX - gestellR, altitude])
|> xLine(length = gestellD)
|> arc(interiorAbsolute = [offsetX, altitude - gestellR], endAbsolute = [offsetX + gestellR, altitude])
|> xLine(length = gestellD)
|> arc(
interiorAbsolute = [
offsetX,
altitude - gestellR - gestellD
],
endAbsolute = [profileStartX(%), profileStartY(%)],
)
|> close()
|> extrude(length = tiefe)
return armBody
}
mirror = mirrorFn(
plane = XZ,
offsetX = armLength,
offsetY = armLength,
altitude = hingeHeight * 4 + hingeGap * 3 + mirrorRadius + archToMirrorGap + archThickness,
radius = mirrorRadius,
tiefe = mirrorThickness,
gestellR = archRadius,
gestellD = archThickness,
)